1. Static의 개념 : 모든 인스턴스에서 공통적으로 사용하는 것
2. Static 변수
class Person{
static int person_count=0; //공유영역
public int age=0; //멤벼변수
public String name; //멤버변수
Person(String param_name){ //생성자, 인자
this.name=param_name;
person_count++;
age++;
}
public void print_info(){
System.out.printIn("인구:" + person_count);
System.out.printIn(name + ":" + age);
}
}
public static void main(String[] args){
Person p1 = new Person("홍길동");
p1.print_info(); //인구:1 홍길동:1
Person p2 = new Person("김길동");
p2.print_info(); //인구:2 홍길동:1
}
public static void main(String[] args){
Person p1 = new Person("홍길동");
p1.print_info(); //인구:1 이름:홍길동
p1.person_count=10;
Person p2 = new Person("김길동");
p2.print_info(); //인구:11 이름:김길동
}
3. Static 메서드
class Person{
static int person_count=0;
public int age=0;
Person(){
person_count++; //1 ->2
}
public static int get_count(){
age=1;
return this.person_count;
}
}
public static void main(String[] args){
Person p1 = new Person();
System.out.printIn(p1.get_count()); //age:1, get_count:1
Person p2 = new Person();
System.out.printIn(p2.get_count()); //age:1, get_count:2
System.out.printIn(p1.get_count()); //age:1, get_count:2
System.out.printIn(Person.get_count()); //static인 경우 객체인 Person 사용가능
//age:1, get_count:2
}
다음 자바 프로그램의 출력값으로 옳은것은?
class Student{
int id;
char name;
static int count=0;
Student(){
count++;
}
}
public class StudentTest{
public static void main(String[] args){
Student man1 = new Student(); //1
Student man2 = new Student(); //2
Student man3 = new Student(); //3
Student man4 = new Student(); //4
}
System.out.printIn(Student.count); //4
}
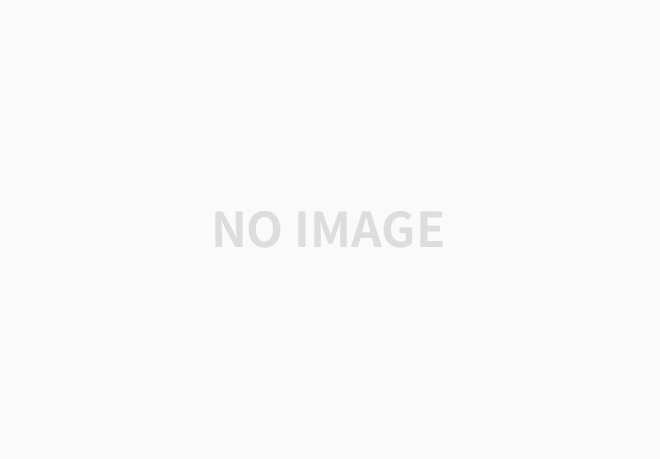
반응형
'정보처리기사 > [흥달쌤] 실기_JAVA 특강 (완)' 카테고리의 다른 글
JAVA특강 / 07. 추상클래스&Interface (0) | 2022.04.29 |
---|---|
JAVA특강 / 05. 접근지정자 (0) | 2022.04.29 |
JAVA특강 / 04. 메서드 오버로딩&오버라이딩 (0) | 2022.04.29 |
JAVA특강 / 03. 상속 (0) | 2022.04.29 |
JAVA특강 / 02. 생성자&예외 (0) | 2022.04.28 |